In software development, testing plays a huge role. It helps developers track and eliminate bugs in the code which makes clients happier and wealthier. Who would like to buy a product full of issues? I can’t really thing of anyone. My colleague sent me a website publishing JS statistics across a couple of years (https://2019.stateofjs.com/). I was looking for a new testing framework when I saw this chart:
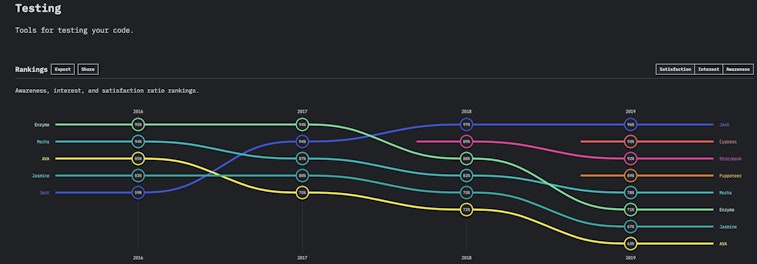
This picture presents the programmers’ satisfaction of using specific test frameworks. I was using Mocha, but I heard a lot of good opinions about Jest. On this screen capture, Jest is on the top of the satisfaction levels so I decided to check it out! In this article, I’m going to describe my experiences from testing Jest.
Jest - First Contact
Once I got to the Jest webpage, the first thing I saw was a cool card animation representing passing/failing tests. The animation gives the website a nice touch and catches user’s attention immediately. But let’s get to the more significant details now!
The philosophy of Jest is that it allows for writing tests with an approachable, familiar and feature-rich API that gives the results quickly. Jest is well-documented, requires little configuration, and can be extended to match our requirements. Sounds good, doesn’t it? Jest is working with projects using Babel, TS, Node, React, Angular, Vue, and more other tech.
I think it’s time to test the test framework in practice!
Setting up the environment
Let’s start from setting up the project. Here, I’m using npm to initialize node project. To initialize a project use command: npm init.
Then I fill the package json file with dev dependencies:
// package.json
{
"name": "typescript-jest-example",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"tests": "jest --config jest-config.json"
},
"repository": {
"type": "git",
"url": "git+https://github.com/DominikMarciniszyn/typescript-jest-example.git"
},
"keywords": [
"jest",
"typescript",
"testing"
],
"author": "Rouch",
"license": "ISC",
"bugs": {
"url": "https://github.com/DominikMarciniszyn/typescript-jest-example/issues"
},
"homepage": "https://github.com/DominikMarciniszyn/typescript-jest-example#readme",
"devDependencies": {
"@types/jest": "22.2.3",
"jest": "26.0.1",
"nodemon": "2.0.4",
"ts-jest": "26.0.0",
"typescript": "3.4.3"
}
}
To get working environment we need to set up the tsconfig and jestconfig. Let’s begin with typescript config:
// tsconfig.json
{
"compilerOptions": {
"target": "ES2019",
"module": "commonjs",
"declaration": true,
"outDir": "./lib",
"strict": true,
"moduleResolution": "node",
"esModuleInterop":true,
"inlineSourceMap": false,
"lib": [
"es2019"
]
},
"include": [
"src"
],
"exclude": [
"node_modules",
"**/tests/*"
]
}
Then jest test framework config:
// jest-config.json
{
"transform": {
"^.+\\.(t|j)sx?$": "ts-jest"
},
"testRegex": "(/tests/.*|(\\.|/)(test|spec))\\.[jt]sx?$",
"moduleFileExtensions": [ "ts", "tsx", "js", "jsx", "json", "node" ],
"collectCoverage": true
}
Now, we should be ready to write some code and test it by using Jest.
Working with the Jest test framework
Let’s start from the basics. We define a simple method that adds two positive numbers. If a number is negative, the method should return an Error with the message ‘Parameters must be greater than zero!’. The method code below:
type NumberOrError = number | Error;
export default class Utils {
addValues(x: number, y: number): NumberOrError {
if (x > 0 && y > 0) {
return x + y;
}
throw new Error('Parameters must be greater than zero!');
}
}
Once we have got our method, we need to test it. First, we check if 1+2 will give us the result that equals to 3. The test code looks like this:
import Utils from '../src/utils';
describe('Utils test case', () => {
it('Add 1 + 2, should return 3', () => {
const utils = new Utils();
const result = 3;
expect(utils.addValues(1, 2)).toBe(result);
});
});
We can run the test with the command: npm run tests. As you remember, we defined the test command in our package json "tests": "jest --config jest-config.json”. When we run the test in the terminal, we should see an interesting output:
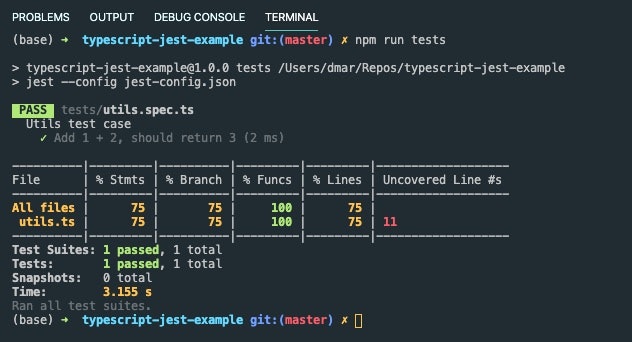
In the picture, we can see which tests were executed. Then we get a table containing the coverage per file. Thanks to our jest-config.json file we are getting the coverage for all our files. The result shows us that our coverage is 75%. And that’s correct — we need to cover the Exception, as well.
it('Add -1 and 2 should return an Error', () => {
const utils = new Utils();
expect(() => {
utils.addValues(-1, 2)
}).toThrow('Parameters must be greater than zero!');
});
Now, when we run the tests, we should see that everything is covered.
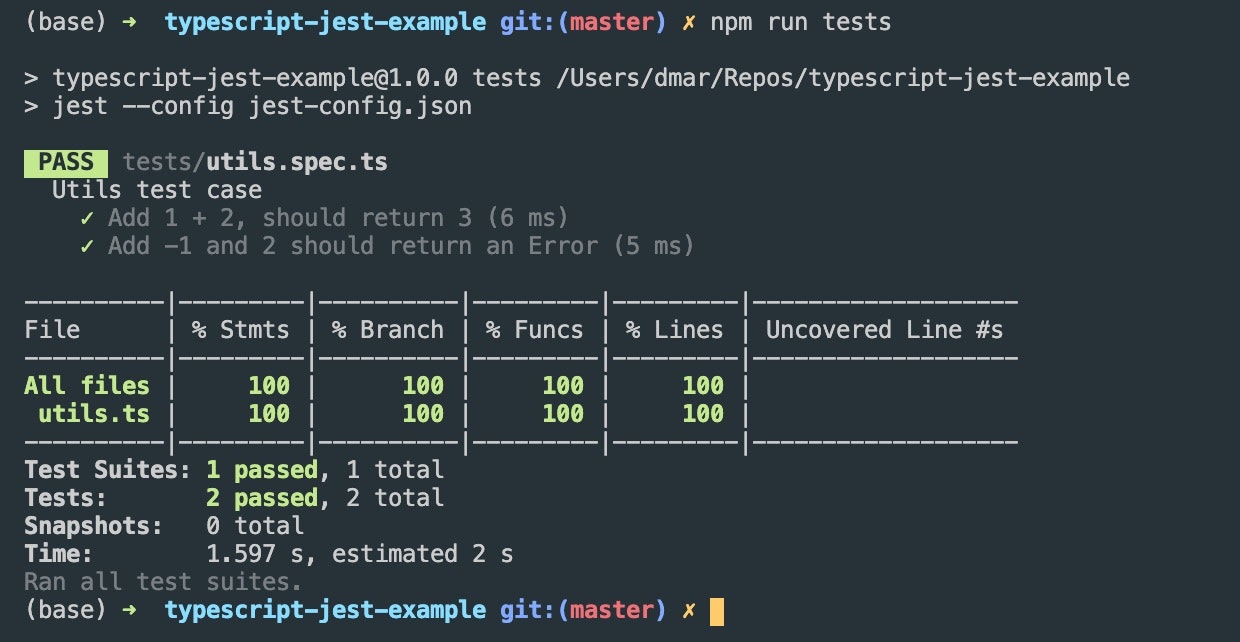
More examples of how to use the Jest test framework you can find in the documentation. The documentation describes topics such as setting async code, setup and teardown, mocks, and more. Additionally, we can find particular guides to, e.g., testing with MongoDB or DynamoDB or specific frameworks like ReactJS or Native apps.
Summary
As you can see, the Jest is a very comfortable testing framework. The thing I didn’t mention before is that migration from other test framework to Jest is also quite easy and it’s described in the documentation, too. Speaking of, the documentation for Jest has many details and a lot of useful resources.
If you’re looking for a test framework for your projects, Jest is definitely worth your attention!
I hope you enjoyed the article and see you next time!
Useful resources: