Introduction
Some time ago we discussed with the team how we can improve the quality of code in our services and what we can do to increase our skills. So, from to time, we had a situation that someone uses a method in the not correct way. I mean too many or too few parameters or invalid types were passed to the method. Of course, it's a not really big problem when someone writes good unit tests because you can detect an error when you will run unit tests. The problem is that if someone will not do it correctly for some reason and changes will be merged to the code repository then you are able to detect an error only when an application is running. And then it's too late because code is deployed. Probably in some cases, it will not work and another bug ticket will wait for us. So the circle closes and we would like to avoid them, so then we looked in the direction of TypeScript.
What is TypeScript?
- an open-source object-oriented programming language
- developed and maintained by the Microsoft
- a superset of JavaScript
- adds optional static typing to the language
- everything that can be written in JavaScript can also be written in TypeScript
Below on the picture, you can see that TypeScript contains all of the functionality of JavaScript and set of own functionalities.
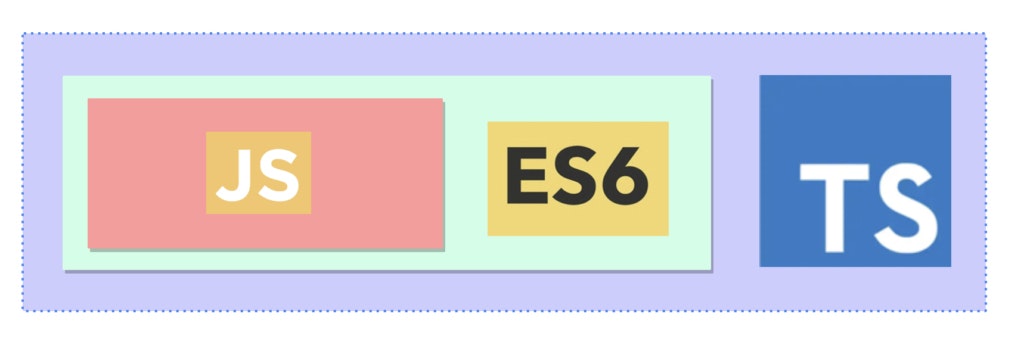
TypeScript compiler read the input, parse it, analyze it, and translate it into JavaScript output.
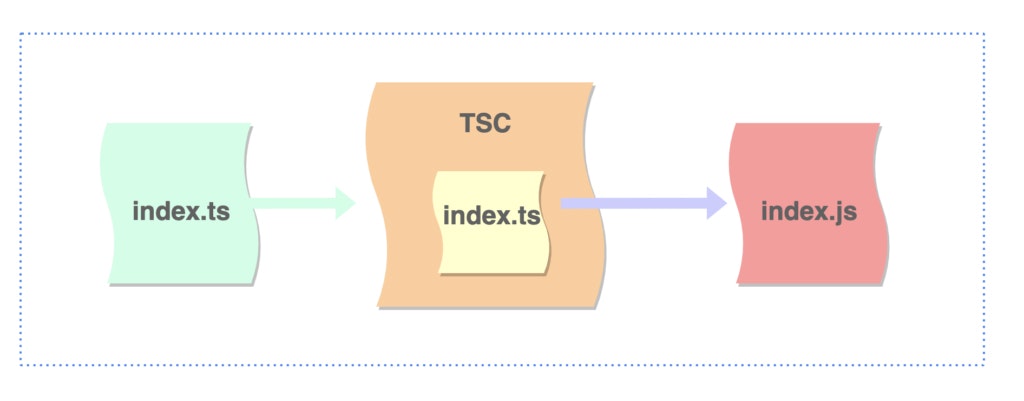
Advantages
Top advantages that make people think about using TypeScript.
- TypeScript simplifies JavaScript code, making it easier to read, debug and understand
- TypeScript allows you to introduce new developers to your project faster
- Static typing is a feature that detects bugs as developers write the code
- ES6 feature support
- Clean code and few bugs due to the point above
- Self-documenting code
- TypeScript helps you dealing with growing teams
- With Dependency Injection, testing becomes easy
- Helps you implementing SOLID design patterns
- More scalable code
- Code completion and IntelliSense
Npm trends
Below on the chart, you can see how the popularity of TypeScript growing up. A lot of big players like Microsoft, Google, Slack, Electron or Asana interest TypeScript and use it in the projects.
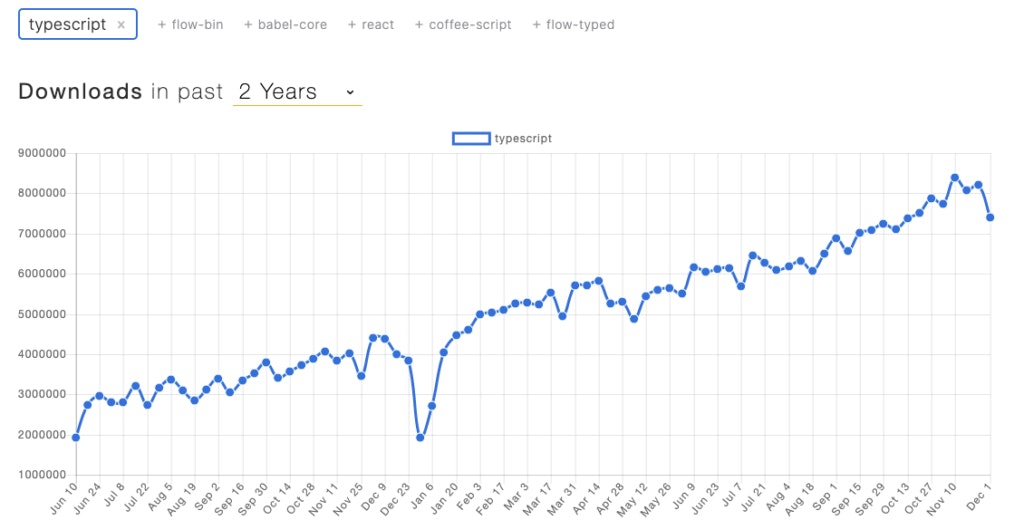
Most important features
Basic types
// boolean - represents the simple true or false value
let isEqual: boolean = false;
// number – represent both Integer as well as Floating-Point numbers
let age: number = 18;
// string – represent a sequence of characters
let name: string = 'JSON';
// array – a type of data structure that stores the elements of similar data type and consider it as an object too
let myTeam: string[] = ['cartman', 'arturito', 'cat', 'father'];
// tuple – enable storing multiple fields of different types in an array with a fixed number of elements
let user: [number, string] = ['Iron', 3];
// enum – allow us to declare a set of named constants i.e. a collection of related values that can be numeric or string values
enum Color {
Red,
Green,
Blue
}
let greenColor: Color = Color.Green;
// any – any type of value can be assigned to that variable
let notSure: any = 4;
notSure = "maybe a string instead";
notSure = false;
Access modifiers
- public – access from the internal class methods as well as from the external scripts
- protected – access from the internal class methods or from its descendants
- private – access from within the class only
Access modifiers control the accessibility of the members of a class. By default, the members are public. For better understanding, the screen below shows the scope of the access modifiers.
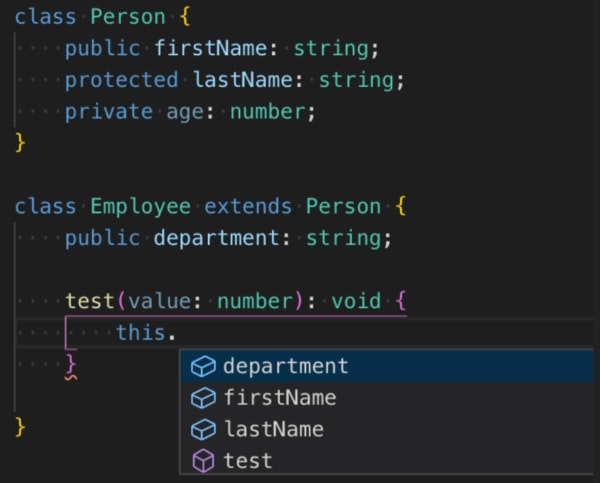
Interfaces
Contains only the declaration of the methods and properties, but not the implementation. It is the responsibility of the class that implements the interface by providing the implementation for all the members of the interface.
interface IPerson {
firstName: string;
lastName: string;
age: number;
}
Error handling
The really interesting thing how TypeScript can improve code quality and reduce the number of bugs is error handling. Below you can see a comparison of error handling in JavaScript and TypeScript.
function askForFood(food: string) {
console.log(`May I please have ${food}?`);
}
First example:
What will happen if we call askForFood(11)?
In JavaScript we will see the following log in the console: May I please have 11?
In TypeScript, we will receive the error at compile time: Argument of type '11' is not assignable to parameter of type 'string'.
Second example:
What will happen if we call askForFood()?
In JavaScript we will see, the following log in the console: May I please have undefined?
In TypeScript, we will receive the error at compile time: Expected 1 argument but got 0.
Conclusion
We are hoping that using TypeScript in our system we can avoid a lot of trivial bugs and we will be able simplifies JavaScript code, making it easier to read, debug and understand. That’s our goal.
In the next article, I would like to present to you with more information about introduction TypeScript in a step-by-step approach.